Deploying a REST API#
Data Science & AI Workbench enables you to deploy your machine learning or predictive models as a REST API endpoint. You can then share your deployment with colleagues and have them query your model as you continue to update, improve, and redeploy as needed.
There are many tools available in the open-source market for building REST APIs within the Python and R ecosystems. This topic explains how to generate a REST API endpoint from a python function you’ve created within a Jupyter Notebook.
Note
REST API endpoints deployed using Workbench are secure and only accessible to users that you’ve shared the deployment with or users that have generated a token that can be used to query the REST API endpoint outside of Workbench.
Install Tranquilizer#
Open a session and run the following command to install Tranquilizer:
anaconda-project add-packages tranquilizer nbconvert
There are three essential skills you must understand in order to use Tranquilizer effectively:
Functions - Defined sections of code that perform specific tasks.
Type Hints - A method to statically indicate the type of a value within your Python code.
Docstrings - Comments in the code that document what a given function does.
Note
Tranquilizer reads the function’s docstring to automatically generate Swagger documentation for the REST API.
Decorate your function#
Decorated functions are identified by the Tranquilizer server. These functions are executed when HTTP requests are made to the server.
Here is an example of a temperature conversion function with the tranquilizer decoration:
from tranquilizer import tranquilize
@tranquilize(method='get')
def to_celsius(fahrenheit: float):
'''Convert degrees Fahrenheit to degrees Celsius
:param fahrenheit: Degrees fahrenheit'''
return (fahrenheit - 32) * 5/9
Note
When the Tranquilizer server starts, the entire Jupyter Notebook is executed. Consider carefully how you arrange operations that are heavy on CPU or memory usage, like reading data or loading models. Computations for reusable objects should be performed only once, rather than every time the function is called.
Add the command#
With tranquilizer downloaded and your function decorated, you must now prepare the deployment command. Open your anaconda-project.yml
file and add the following code:
commands:
celsius_api:
unix: tranquilizer converter.ipynb --name "Temperature Conversion"
supports_http_options: True
Caution
Make sure your notebook file name is spelled correctly! If it is not, your commands will not work.
Alternatively, you can run the following command in the terminal to add the command to the anaconda-project.yml file:
anaconda-project add-command --type unix --supports-http-options celsius_api "tranquilizer converter.ipynb --name 'Temperature Conversion'"
Commit and deploy#
Commit all changes to your project and deploy the command. Choose a unique URL for your deployment.
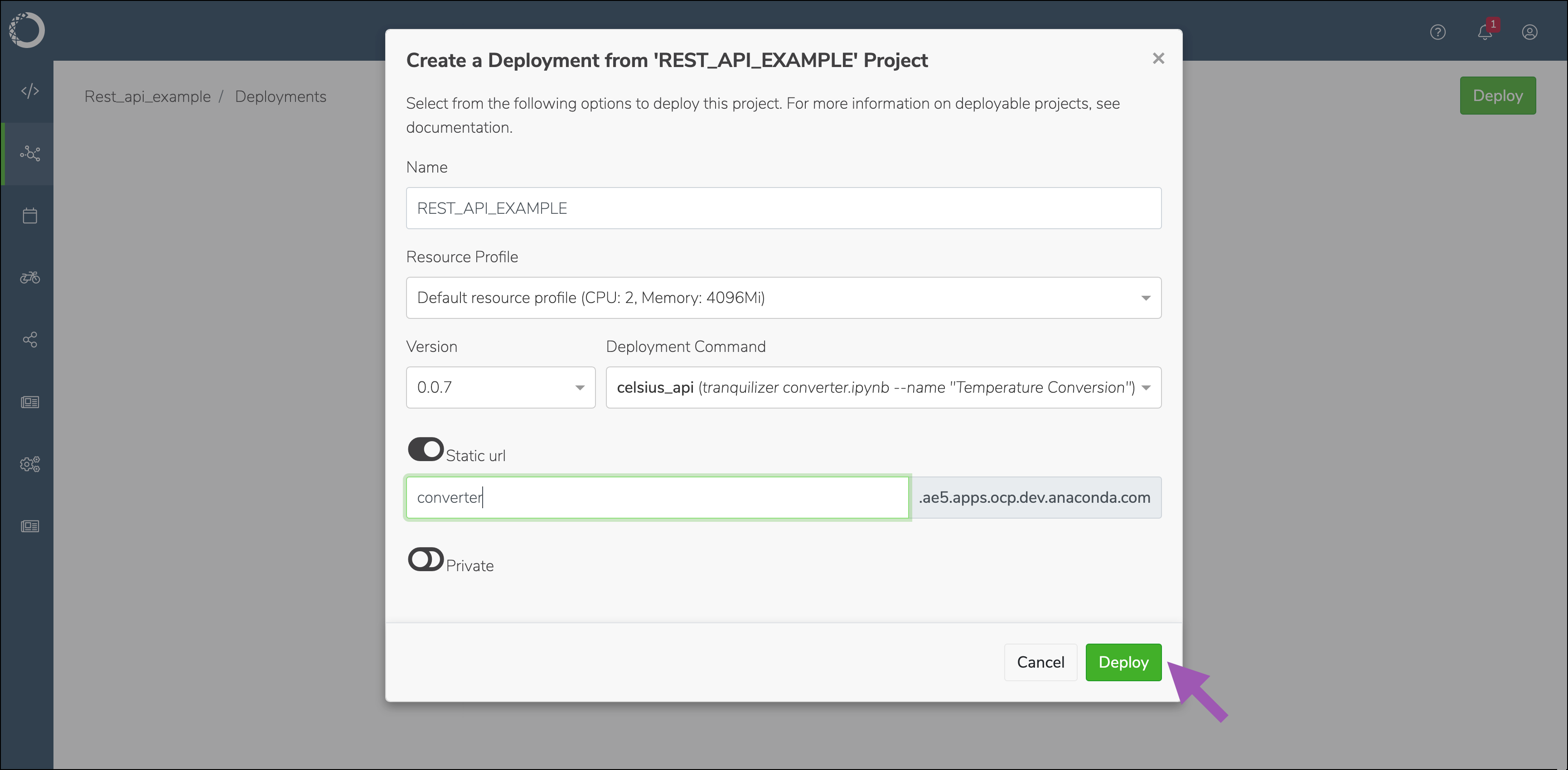
Verify deployment#
Once the deployment has started, go to the deployments View page and use swagger to verify that the API performs the correct function.
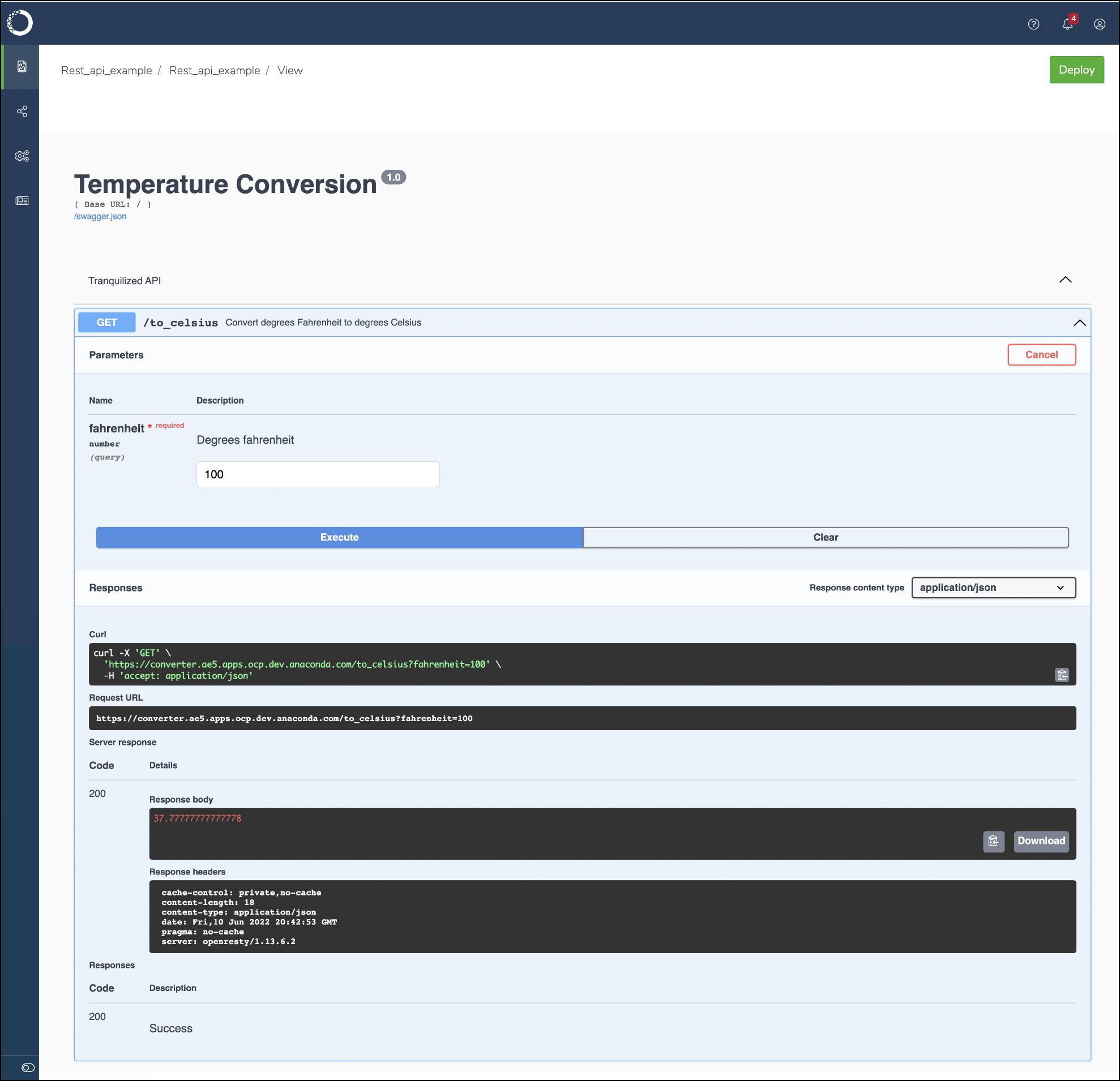
Deploy a REST API with R#
You can use the R Plumber package to build and deploy a REST API in R.
Run the following command to install R Plumber
:
anaconda-project add-packages -c r r-plumber
The API is defined in a file called api.R
library(plumber)
#* @apiTitle Temperature Converter
#* Convert degrees Fahrenheit to Celsius
#* @param fahrenheit:numeric Degrees Fahrenheit
#* @get /to_celsius
function(fahrenheit) {
(as.numeric(fahrenheit) - 32) * 5/9
}
#* Redirect to Swagger documentation
#* @get /
function(res) {
res$body <- "<html><meta http-equiv='refresh' content=\"0; URL='/__swagger__/'\" /></html>"
res
}
To separate your code into api.r
and run.R
scripts, add your command specification in the anaconda-project.yml
file:
commands:
api:
unix: Rscript run.R
Then format your run.R
script as follows:
plumber::plumb("api.R")$run(port = 8086, host = '0.0.0.0', swagger = TRUE)
Alternatively, you can place the contents of the the run.R
script directly in the anaconda-project.yml
file:
commands:
api:
unix: R -e 'plumber::plumb("api.R")$run(port = 8086, host = "0.0.0.0", swagger = TRUE)'